- Today
- Total
작심삼일
[LeetCode] 39 | Combination Sum | Python 본문
문제 링크: https://leetcode.com/problems/combination-sum/
문제
Given an array of distinct integers candidates and a target integer target, return a list of all unique combinations of candidates where the chosen numbers sum to target. You may return the combinations in any order.
The same number may be chosen from candidates an unlimited number of times. Two combinations are unique if the frequency of at least one of the chosen numbers is different.
It is guaranteed that the number of unique combinations that sum up to target is less than 150 combinations for the given input.
조건
- 1 <= candidates.length <= 30
- 1 <= candidates[i] <= 200
- All elements of candidates are distinct.
- 1 <= target <= 500
내 풀이
candidates중에 target보다 큰 것을 제외한 후 내림차순으로 정렬한다.
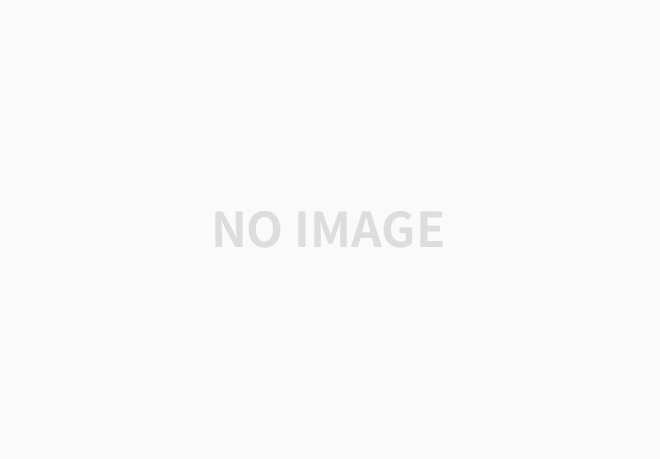
그 후, 맨 앞에서부터 차례로 더해보는 make_comb라는 함수를 이용해 문제를 풀었다.
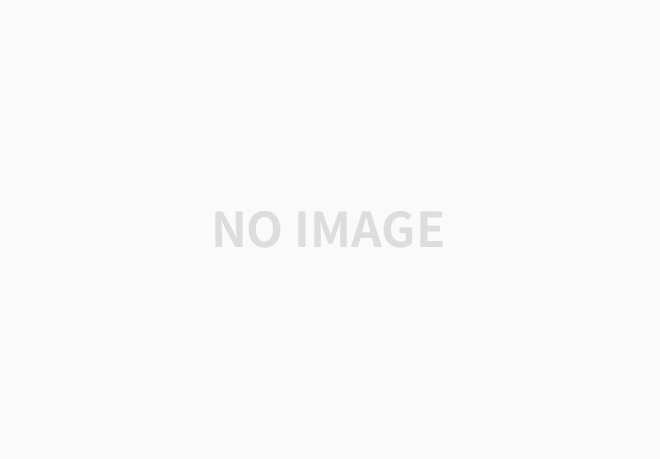
comb 내의 합이 7이니 정답에 추가
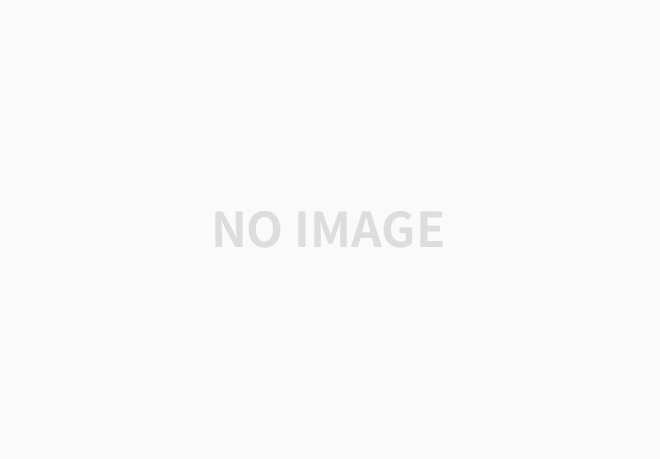
6으로 시작하는 comb들로는 합을 7로 만들 수 없다.
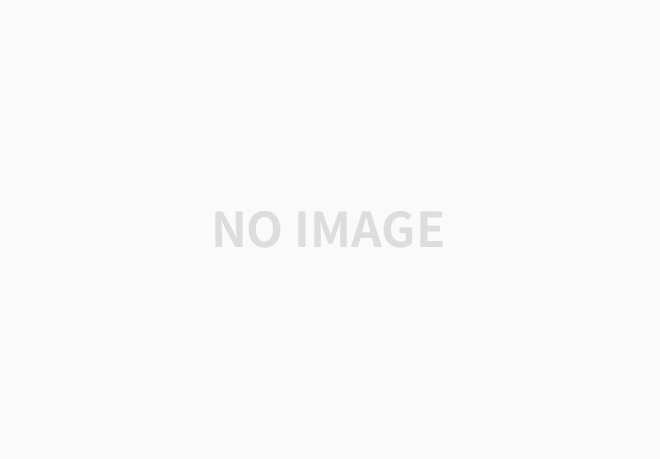
3으로 시작하는 comb중에서는 [3, 2, 2]의 합이 7이 된다.
이를 반복하면 된다.
코드
class Solution:
def combinationSum(self, candidates: List[int], target: int) -> List[List[int]]:
self.candidates = sorted(list(filter(lambda x: x <= target, candidates)), reverse=True)
self.target = target
self.N = len(self.candidates)
self.ans = []
if self.N == 0:
return []
self.make_comb(0, 0, [])
return self.ans
def make_comb(self, start, cur_sum, comb):
for n in range(start, self.N):
if cur_sum + self.candidates[n] < self.target:
comb.append(self.candidates[n])
self.make_comb(n, cur_sum+self.candidates[n], comb)
del comb[-1]
elif cur_sum + self.candidates[n] > self.target:
continue
elif cur_sum + self.candidates[n] == self.target:
comb.append(self.candidates[n])
self.ans.append(comb[:])
del comb[-1]
'스터디 > 코테' 카테고리의 다른 글
[LeetCode] 169 | Majority Element | Python (0) | 2022.02.21 |
---|---|
[LeetCode] 402 | Remove K Digits | Python (0) | 2022.02.18 |
[LeetCode] 24 | Swap Nodes in Pairs | Python (0) | 2022.02.16 |
[LeetCode] 136 | Single Number | Python (0) | 2022.02.15 |
[LeetCode] 560 | Subarray Sum Equals K | Python (0) | 2022.02.10 |